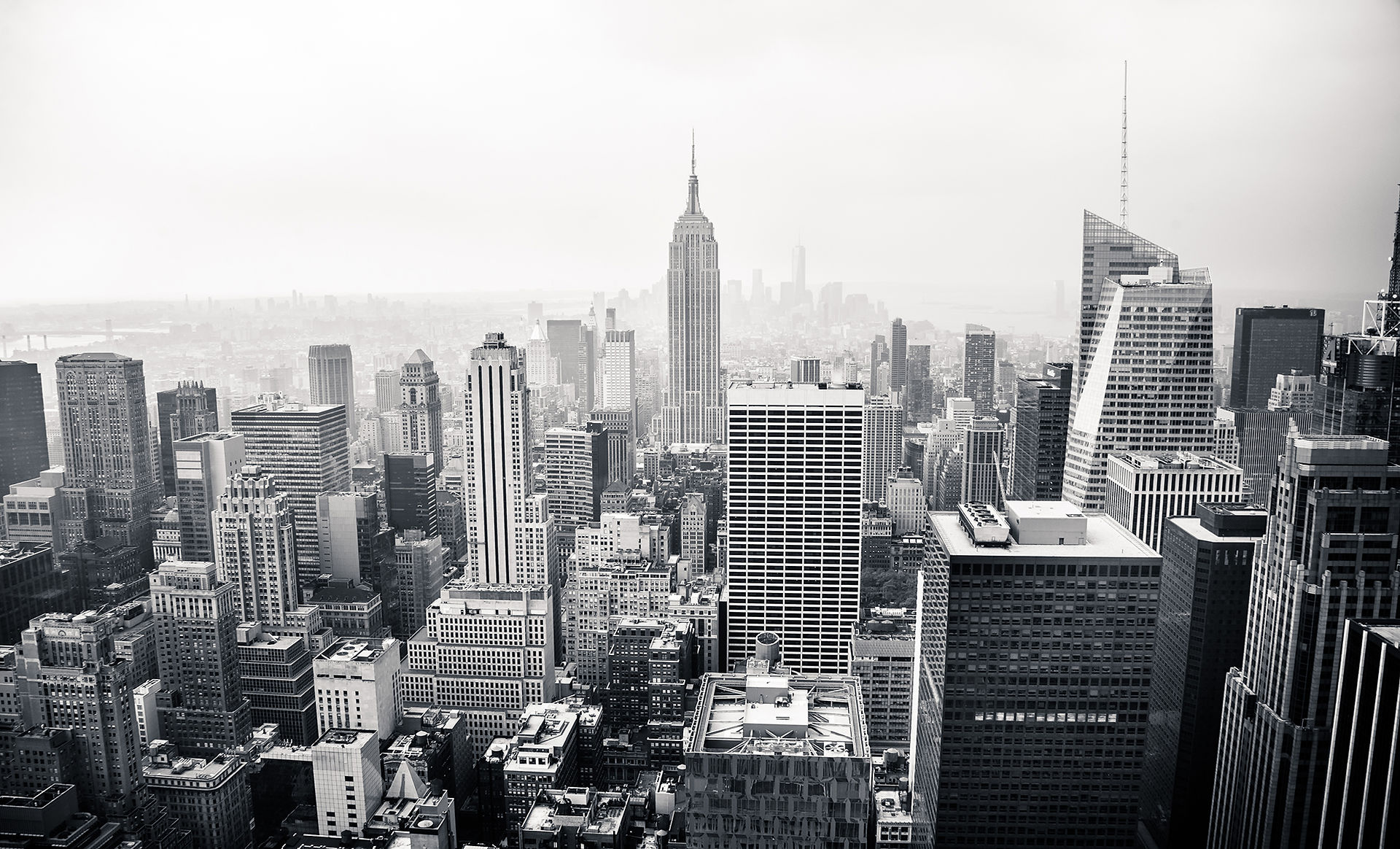
BMI Tracker: Android Application Development
As part of my second year module, I created an application for Android that is used to calculate and track ones BMI, which is stored within a database. The reason behind creating this specific application is that having an application to easily store one’s body mass index on a convenient mobile platform means people will be more likely to continually record and monitor this attribute, which will potentially lead to a greater interest in health and fitness.
Market Research
From looking at the Google play store, I found that other such applications exist presently for android, each with altering levels of functionality. In order for my application to I decided to look into a few of the existing applications that focus mainly on the calculation aspect of BMI monitoring:
1. ‘Ideal Weight (BMI)’, mmapps mobile
Found at: https://play.google.com/store/apps/details?id=mmapps.bmi.calculator
The user is able to enter their details to calculate their BMI, and have their ‘ideal weight’ returned. However, I feel that for one screen on the app, there is far too much content; the user is overloaded with information. The solution to this would be to split this up onto multiple pages.
2. ‘Body Mass Index Calculator’, JSU Developers
Found at: https://play.google.com/store/apps/details?id=com.jsu.first.media.BMI
This application consist of one page which has the calculator, with the option to change the units for the user entry. The user interface is simple, but intuitive, with aspects such as the arrows within the text entry fields, indicating that there contains extra functionality.
Design
After looking into the current applications of this function, I started designing the interface for my own application, using fluid (Fluid UI 2015). Initially for my application, I wanted to have a basic menu page with buttons to link to different activities, as shown in figure 3:
From there, the user can visit any of the three pages: BMI Calculator, Enter BMI, or BMI History.
The BMI Calculator page would contain two text entry fields for the user to enter their weight and height; the two attributes needed to calculate ones BMI. Below this, there would be a text field that would show the calculated BMI, based on the user’s input, as shown in figure 4:
The Enter BMI page has 3 text fields: date, weight, BMI. The user would enter their details, and could save it by clicking the save button. The data would then be saved into a database, which they would be able to access on the BMI History page. The load button would allow the user to load the last saved entry, had they closed the app but wished to load their last entry. This is shown is figure 5:
The final page allows the user to look at their past entries that they have saved, shown in figure 6. They could click on an entry of their choice, and view the details recorded on that day. After designing the basic functionality of the application, I began the implementation using Android Studio.
Implementation and Features
The end result of my implementation is shown below in figures 7 & 8. The app consists of the following ‘pages’: a BMI entry page, a BMI Calculator.
Interface:
Following the Android Design Principles, I created the application with a clear and intuitive layout. Its minimal design ensures the ‘Keep it brief’ requirement is met, which specifies that ‘short phrases and simple words’ should be used. The ‘If it looks the same, it should act the same’ principle has been put into practice inspired the button design; all buttons follow the same design, indicating that each of them work in the same way. This also follows the Gestalt principle of similarity; when objects appear alike, it is assumed they follow the same pattern (Graphicdesign.spokanefalls.edu 2015).
In each of the text entry fields in the BMI Entry page, there is a ‘hint’, informing the user of what data needs to be entered. This is created in the XML file for MainActivity.
Activities:
In my application I created two activities: the BMI Entry page and the BMI Calculator page. The first activity (MainActivity) contains methods for creating the database to store the data, saving the data to the database, and loading the data again. These methods are discussed further in the repository code, which can be provided upon request.
Data Persistence:
When the application is closed fully, the data previously entered remains within the interface, so if the user accidently closes the application then their data will not be lost. All the data saved within the application can be viewed on the Android Device Monitor, shown below in figures 9, 10 and 11:
SQLite Database:
I also implemented and SQLite database within the application. When the user enters the date, their weight, and BMI, and click the save button, the information is put into a SQLite database. Again, the database can be accessed using the Android Device Monitor, shown in figure 12. After the file is extracted, it can be viewed fully by using SQLiteStudio, which is an open source database manager (Sqlitestudio.pl 2015).
Database Handler:
A separate class was created to create the SQLite database, which will have data inserted into it in the main activity. Three columns are defined for the date, weight, and BMI. The addRecord method is responsible for inserting data into the database, using the get methods defined within the Record class. If the data has been inserted correctly, then this is declared within the Log, shown in figures 13 and 14:
Calculator:
In the second activity, there is a calculator that shows the user their BMI based on their current weight and height. The user inputs their height and weight and the result is inserted into the text field. This is done by dividing the weight in kilograms by the height in centimetres squared, multiplied by 10,000. The calculator was based on the implementation by JejjE's network, 2015.
The calculator activity is accessed by using intents. An intent is an object that components in the app use to communicate with the operating system. I am using an explicit intent, as opposed to an implicit intent, as explicit intents are used to start activities in within the application; implicit intents are used to request another application on the device to carry out a certain action.
Toasts:
In the first activity, when the user clicks a button, a pop up message, or a ‘Toast’ appears. A toast is a message that is used to inform the user that an activity has been carried out. For example, when the user clicks the save button, a toast appears, giving the user feedback that their action was successful (see figure 16). In the second activity, a toast appears when the user attempts to calculate their BMI without entering either their height or weight. This informs the user that the corresponding field must be filled out.
Future Development
Although the application has the basic functionality I wanted, there features that I would like to implement in the future. For example, in the design I set myself, I would have liked to implement an activity which included all of the CRUD principles for the database, where the user would be able to read, update, and delete entries. I would also like to implement the main menu screen, from which the user would be able to select any of the activities and go there straight away, rather than having to go through other activities.
An extra feature I would have like to implement would have been a separate activity that would contain a graph that would plot the user’s weight and BMI overtime. The graph would pull the data from the SQLite database. This could be implemented using the GraphView library, created for Android Studio. The library allows you to plot a variety of charts, including line graphs, which I would aim to use. This would provide the user with additional visual feedback on their entries.
References
Fluid UI, (2015) Fluid UI - Free Web And Mobile App Prototyping [online] available from <https://www.fluidui.com/> [14 December 2015]
Principles, A. (2015) Android Design Principles | Android Developers [online] available from <http://developer.android.com/design/get-started/principles.html#simplify-my-life> [10 December 2015]
Graphicdesign.spokanefalls.edu, (2015) The Gestalt Principles [online] available from <http://graphicdesign.spokanefalls.edu/tutorials/process/gestaltprinciples/gestaltprinc.htm> [17 December 2015]
Sqlitestudio.pl, (2015) Sqlitestudio [online] available from <http://sqlitestudio.pl/> [15 December 2015]
Anon. (2015) Graph View [online] available from <http://www.android-graphview.org/> [17 December 2015]
Developer.android.com, (2015) Starting An Activity | Android Developers [online] available from <http://developer.android.com/training/basics/activity-lifecycle/starting.html#Create> [15 December 2015]
How, T. (2014) Android Studio Sqlite Database Example [online] available from <http://instinctcoder.com/android-studio-sqlite-database-example/> [15 December 2015]
Developer.android.com, (2015) Starting Another Activity | Android Developers [online] available from <http://developer.android.com/training/basics/firstapp/starting-activity.html#DisplayMessage> [10 December 2015]
Play.google.com, (2015) available from <https://play.google.com/store/apps/details?id=mmapps.bmi.calculator> [7 December 2015]
Play.google.com, (2015) available from <https://play.google.com/store/apps/details?id=com.jsu.first.media.BMI> [7 December 2015]
JejjE's network, (2015) Make Your Own BMI Calculator Android App - Jejje's Network [online] available from <http://jejje.net/make-your-own-bmi-calculator-android-app/> [16 December 2015]
Phillips, B., Hardy, B., Stewart, C. and Marsicano, K. (n.d.) Android Programming.
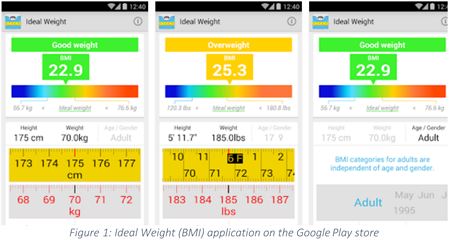

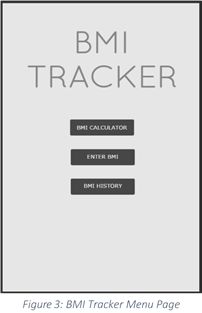
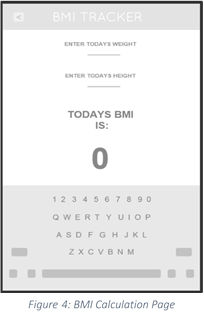

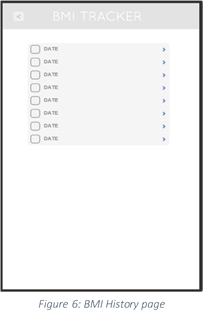
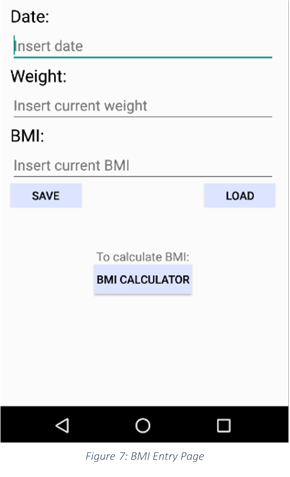


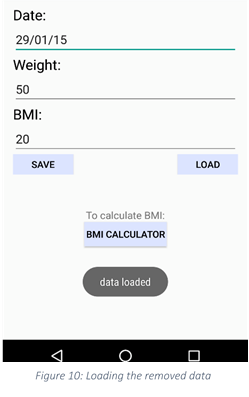

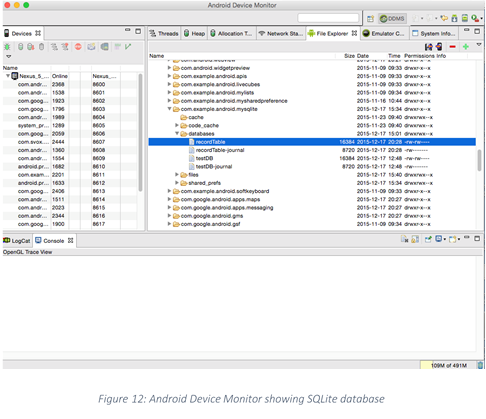
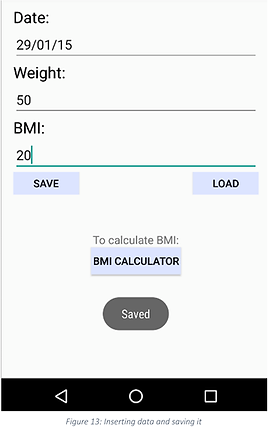
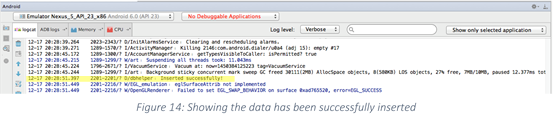
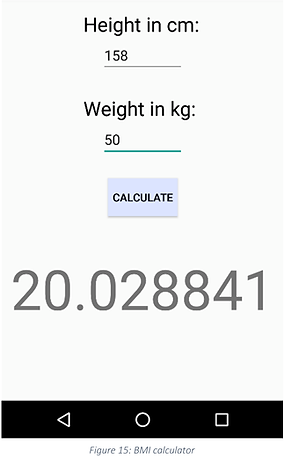
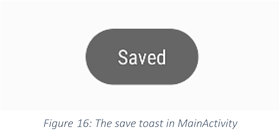
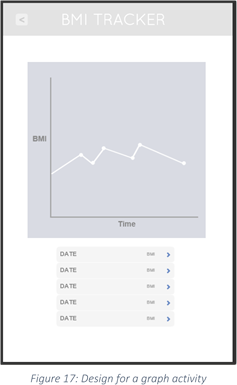